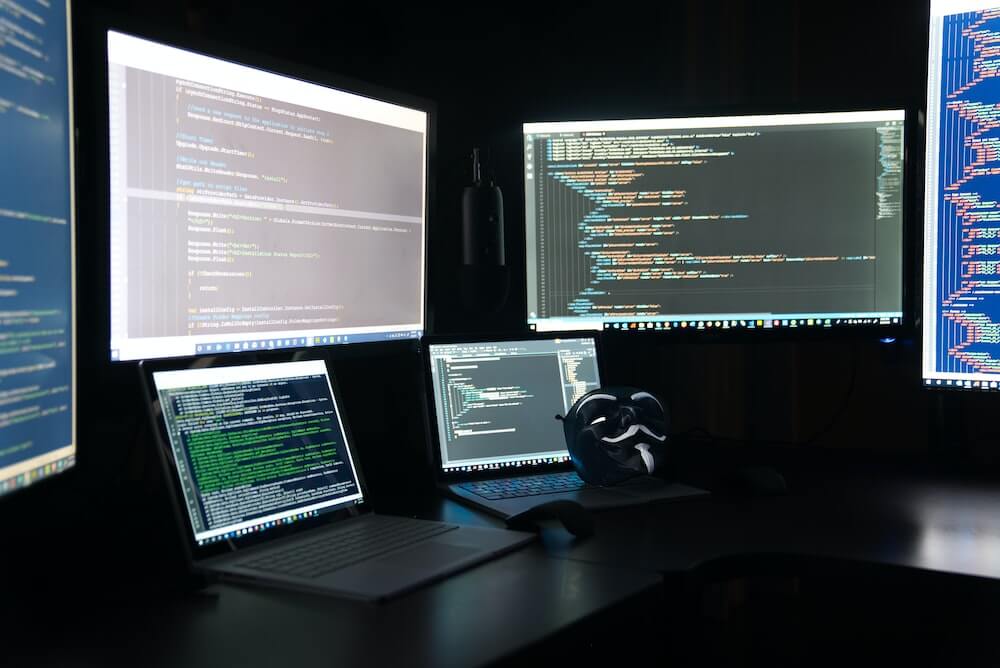
Understanding RESTful APIs: A Beginner’s Guide
Introduction to APIs
In today’s digital world, Application Programming Interfaces (APIs) are the backbone of data exchange between software applications. APIs allow different software systems to communicate with each other, enabling them to work together to provide a seamless user experience. Whether you’re booking a flight, checking the weather on your phone, or using a social media app, APIs are working behind the scenes to make these interactions possible.
What is a RESTful API?
REST (Representational State Transfer) is an architectural style for designing networked applications. A RESTful API is an API that adheres to the principles of REST. It uses HTTP requests to perform CRUD (Create, Read, Update, Delete) operations on resources. These resources are typically represented in JSON (JavaScript Object Notation) or XML (eXtensible Markup Language) format.
Core Principles of REST
- Statelessness: Each HTTP request from a client to server must contain all the information the server needs to fulfill that request. The server does not store any information about the client state between requests.
- Client-Server Architecture: The client and server are separate entities. The client is responsible for the user interface, while the server handles the backend and data storage. This separation allows both to evolve independently.
- Cacheability: Responses from the server should be explicitly marked as cacheable or non-cacheable. This can improve the efficiency and performance of the client-server interaction.
- Layered System: REST allows for an architecture composed of hierarchical layers. Each layer provides a specific functionality, which can enhance scalability and manageability.
- Uniform Interface: This simplifies and decouples the architecture, which allows each part to evolve independently. The key constraint here is that the interface between client and server should be uniform.
Building a Simple RESTful API
Let’s walk through building a simple RESTful API using Express.js, a popular Node.js framework.
Step 1: Setting Up Your Project
First, ensure you have Node.js and npm (Node Package Manager) installed. Then, create a new project directory and initialize a new Node.js project:
bash
Copy code
mkdir rest-api-example
cd rest-api-example
npm init -y
Step 2: Installing Dependencies
Next, install Express.js:
bash
Copy code
npm install express
Step 3: Creating the Server
Create a new file called server.js and set up a basic Express server:
javascript
Copy code
const express = require(‘express’);
const app = express();
const PORT = 3000;
app.use(express.json());
app.get(‘/’, (req, res) => {
res.send(‘Hello, World!’);
});
app.listen(PORT, () => {
console.log(`Server is running on port ${PORT}`);
});
Run the server with:
bash
Copy code
node server.js
Step 4: Defining Routes
Now, let’s define some routes to perform CRUD operations on a simple resource, like books.
javascript
Copy code
let books = [
{ id: 1, title: “1984”, author: “George Orwell” },
{ id: 2, title: “To Kill a Mockingbird”, author: “Harper Lee” }
];
app.get(‘/books’, (req, res) => {
res.json(books);
});
app.get(‘/books/:id’, (req, res) => {
const book = books.find(b => b.id === parseInt(req.params.id));
if (!book) return res.status(404).send(‘Book not found’);
res.json(book);
});
app.post(‘/books’, (req, res) => {
const book = {
id: books.length + 1,
title: req.body.title,
author: req.body.author
};
books.push(book);
res.status(201).json(book);
});
app.put(‘/books/:id’, (req, res) => {
const book = books.find(b => b.id === parseInt(req.params.id));
if (!book) return res.status(404).send(‘Book not found’);
book.title = req.body.title;
book.author = req.body.author;
res.json(book);
});
app.delete(‘/books/:id’, (req, res) => {
const book = books.find(b => b.id === parseInt(req.params.id));
if (!book) return res.status(404).send(‘Book not found’);
const index = books.indexOf(book);
books.splice(index, 1);
res.json(book);
});
Best Practices for Designing RESTful APIs
- Use Nouns for Resource URLs: URLs should represent resources rather than actions. For example, use /books rather than /getBooks.
- Statelessness: Each request from the client to the server must contain all the information the server needs to fulfill that request.
- Proper Use of HTTP Methods:
- GET: Retrieve resources.
- POST: Create a new resource.
- PUT: Update an existing resource.
- DELETE: Remove a resource.
- Use HTTP Status Codes Appropriately: For example, return 404 for not found, 201 for resource creation, and 400 for bad requests.
- Data Validation: Validate input data to ensure the API behaves as expected.
- Versioning: Use versioning in your API URLs to handle changes without breaking existing clients. For example, /api/v1/books.
By adhering to REST principles and best practices, you can create scalable, maintainable, and efficient APIs that serve as the backbone for your applications. With tools like Express.js, building a RESTful API becomes a straightforward and rewarding process. Happy coding!
Leave a comment: